FactoryGirl (Bot) - create vs build vs build_stubbed
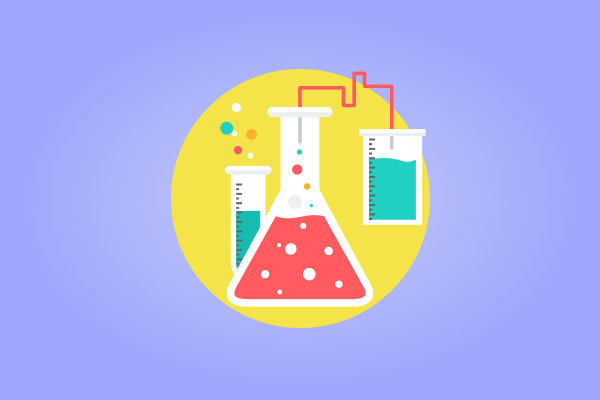
If you are new to writing RSpec tests in Rails using FactoryGirl (bot), you can ask yourself what is the difference between create, build and build_stubbed. In this article, we will do our best to help you understand them better.
Let's say we are testing a valid profile creation:
FactoryBot.define do
factory :profile do
user
sequence(:name) { |n| "Name#{n}" }
end
end
describe Profile, type: :model do
it 'creates valid profile' do
expect(FactoryBot.create(:profile)).to be_valid
end
end
FactoryBot.create(:profile)
will create a profile object and all associations for it. They will all be persisted in the database. Also, it will trigger both model and database validations. Example test performance with create is:
Note: after(:build) and after(:create) will be called after the factory is saved. Also, before(:create) will be called before factory is saved.
On the other hand, FactoryBot.build(:profile)
won't save the object, but will still make requests to a database if the factory has associations. It will trigger validations only for associated objects. Example test performance with build is:
Note: after(:build) will be called after factory is built.
FactoryBot.build_stubbed(:profile)
does not call database at all. It creates and assigns attributes to an object to make it behave like an instantiated object. It provides a fake id and created_at. Associations, if any, will be created via build_stubbed too. It will not trigger any validations. Example test performance with build_stubbed is:
Note: after(:stub) will be called after factory is stubbed via buildstubbed.
Keep in mind that this was a very simple test example. Better performance results are expected with more complex test suites.
Thank you for reading this article. Hopefully, it helped you better understand the differences between the ways to instantiate the objects when testing with FactoryBot.
If you liked this article please subscribe to our newsletter for more Rails content!
Interested in similar topics? Be sure to check our take on Rspec let vs before. Also, if you're totally new to RSpec, we have a guide to set it up.