Sass Mixins
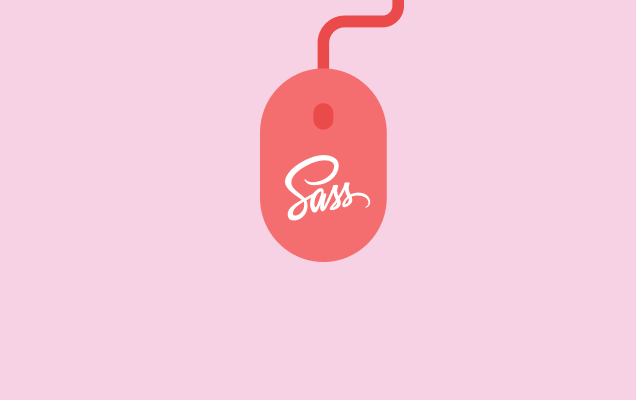
This is the third post about specific Sass features and this one is about mixins. Previous were import feature and extend feature and there was one about nesting in general.
This feature is used in specific situations, not too often but it's too necessary when you master it.
@mixin
To define this feature type @mixin
and the name of your creation. After that, in CSS style - within {}
, type all you want to be defined with it.
@mixin creation-name{
property1:value;
property2:value;
property3:value;
}
When it is defined, you should use it somewhere...To call the mixin feature, type @include
followed by the mixin name inside of selector.
selector{
@include creation-name;
}
After compiling this code into CSS, it will look like this:
selector{
property1:value;
property2:value;
property3:value;
}
Flexible Mixin
A mixin lets you pass in an argument to make it flexible. This is defined by adding the value after the name of the mixin. That value can be used inside of mixin (as much time as you need).
@mixin creation-name($value){
property1:$value;
property2:value;
property3:value;
}
After mixin is defined this way when it's called after @include
and the creation name you must forward that value to.
selector{
@include creation-name(10px);
}
After compiling...
selector{
property1:10px;
property2:value;
property3:value;
}
You can add an infinite number of values inside of ()
separated with ,
Where to use it
Now when you understand what it is about, you must feel that there are specific cases for it. Everyone who works with Sass (and uses it correctly) has his own collection and this is mine:
Pseudo
When using ::before
and ::after
you'll always need these three, so we're saving two lines of code every time you use this.
@mixin pseudo{
content: '';
display: block;
position: absolute;
}
Position Center
Block horizontal and vertical centering helper (Important: you must have a parent element with position: relative
).
@mixin center {
position:absolute;
top:50%;
left:50%;
-webkit-transform: translate(-50%, -50%);
-ms-transform: translate(-50%, -50%);
transform: translate(-50%, -50%);
}
Placeholders
I still believe that the mixin is invented for us to use it for browsers' prefixes. This is just one example of that.
@mixin input-placeholder {
&:-moz-placeholder { @content; }
&::-moz-placeholder { @content; }
&:-ms-input-placeholder { @content; }
&::-webkit-input-placeholder { @content; }
}
Breakpoints
You can use breakpoints or media queries but either way, you're going to like this. In this example, @content
mixin feature is used. You can understand from the example that this feature allows adding CSS inside of @include
.
@mixin bp-large {
@media only screen and (max-width: 60em) {
@content;
}
}
@mixin bp-medium {
@media only screen and (max-width: 40em) {
@content;
}
}
@mixin bp-small {
@media only screen and (max-width: 30em) {
@content;
}
}
How to use it:
.sidebar {
width: 60%;
float: left;
@include bp-small {
width: 100%;
float: none;
}
}
Here's some other colections, you can find a lot of interesting things in:
Andy.scss, bourbon, npm, and if you want the advanced option of the mixin feature check this post.
Sum up
Make your collection and mixin it up! (Actually, just copy/paste it into every new Sass project)
For faster access to courses on these topics, subscribe to our newsletter.