Creating Rails Modals Using Bootstrap
Instead of writing your own JavaScript code for handling remote modals in your Rails application, it is a much simpler approach to implement it by using Bootstrap. In this tutorial, we will explain how to do it efficiently.
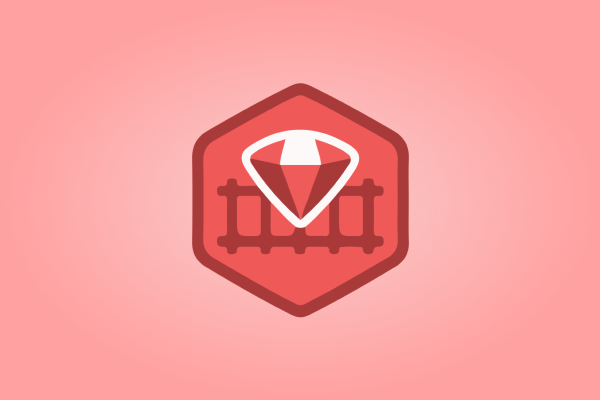
Instead of writing your own JavaScript code for handling remote modals in your Rails application, it is a much simpler approach to implement it by using Bootstrap. In this tutorial, we will explain how to do it efficiently.
Step 1: Initial Setup
Add gem 'bootstrap' and gem 'popperjs' to your Gemfile and run bundle install
.
After installing the gems, update your application.js in the following order:
//= require jquery
//= require popper
//= require turbolinks
//= require bootstrap
//= require_tree.
Step 2: Modify Layouts in View
Make sure that this view is partial. Inside it, you will have the content you want to show in the modal.
_new.html.erb
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">x</button>
<h4 class="modal-title" id="myModalLabel">Modal title</h4>
</div>
<div class="modal-body">
*Modal content comes here*
</div>
<div class="modal-footer">
<button class="btn btn-primary">Save</button>
</div>
We need to define a place where modals will be rendered:
index.html.erb
<%= link_to 'Add user', new_user_path, {:remote => true, 'data-toggle' => "modal", 'data-target' => '#modal-window', class: 'btn btn-primary btn-lg'} %>
<div id="modal-window" class="modal hide fade" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content"></div>
</div>
</div>
Remote is used to tell jquery to submit this form with ajax, while 'data-toggle' => "modal" is used to tell our script to handle this form as the modal form. < div > loads the partial as a modal window and with class="modal hide fade" you can add an extra fade effect to the modal, too! All that is left is to position the modal the way you want.
To make this all work we need to add some JavaScript:
new.js.erb
$("#modal-window").find(".modal-content").html("<%= j (render 'new') %>");
$("#modal-window").modal();
Step 3: Modify Your Controller
In the controller add the respond_to block to be able to use Ajax:
def new
respond_to do |format|
format.html
format.js
end
end
Step 4: Enjoy!
We have finally reached the end! Now you just need to click on "Add user" button and a nice modal will show up with a fade effect! The only thing that might be left is to add some Model and Controller tests, be sure to check how to get started with RSpec and FactoryGirl!
This is our final result:
Thank you for staying with us until the end.
If you want to read more about Rails, be sure to subscribe to our newsletter!