Elastic Search on Rails
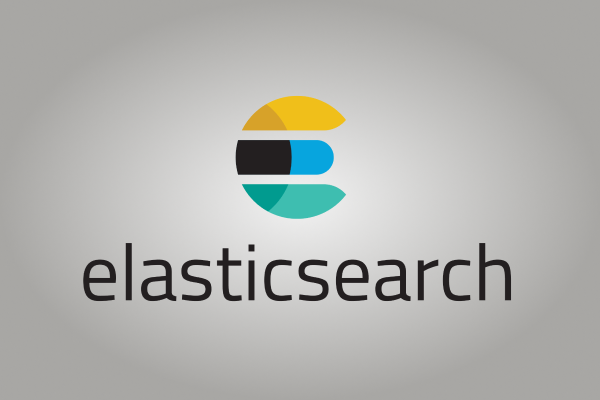
Elastic Search is a mirror DB with indexes of your existing DB, which is searchable trough JSON API. In order to perform the search you will need to have the data synced between your DB and Elastic Search. This can be done via rivers to pull data from your application or to push data to your ES node via _bulk operations.
But let’s start from beginning:
Download Elastic Search deb
cd /usr/share/elasticsearch
# To install Marvel inside development enviroment
sudo bin/plugin -i elasticsearch/marvel/latest
# In same directory run ES
bin/elasticsearch -d
#Check if it's working
localhost:9200
Keeping data in sync
The simple way:
class Article
include Elasticsearch::Model
include Elasticsearch::Model::Callbacks
end
The more complex way would be:
# 1. Bind callbacks
after_commit on: [:create] do
index_document if self.published?
end
after_commit on: [:update] do
update_document if self.published?
end
after_commit on: [:destroy] do
delete_document if self.published?
end
Using async commands – a way to go
Please refer to
https://github.com/elasticsearch/elasticsearch-rails/tree/master/elasticsearch- model
JDBC plugin for Elastic Search
The Java Database Connection (JDBC) plugin allows fetching data from JDBC sources for indexing into Elastic Search.
https://github.com/jprante/elasticsearch-river-jdbc
You can setup a script that pulls data from MySQL into ES. You will have to write custom SQL queries to do so.
Marvel Plugin
#Check Marvel plugin
http://any-server-in-cluster:9200/_plugin/marvel/.
Java versions
We recommend installing the Java 8 update 20 or later, or Java 7 update 55 or later. Previous versions of Java 7 are known to have bugs that can cause index corruption and data loss.
Hosted solutions
https://qbox.io/
Some references:
http://serverfault.com/questions/386557/how-to-index-mysql-from-elasticsearch